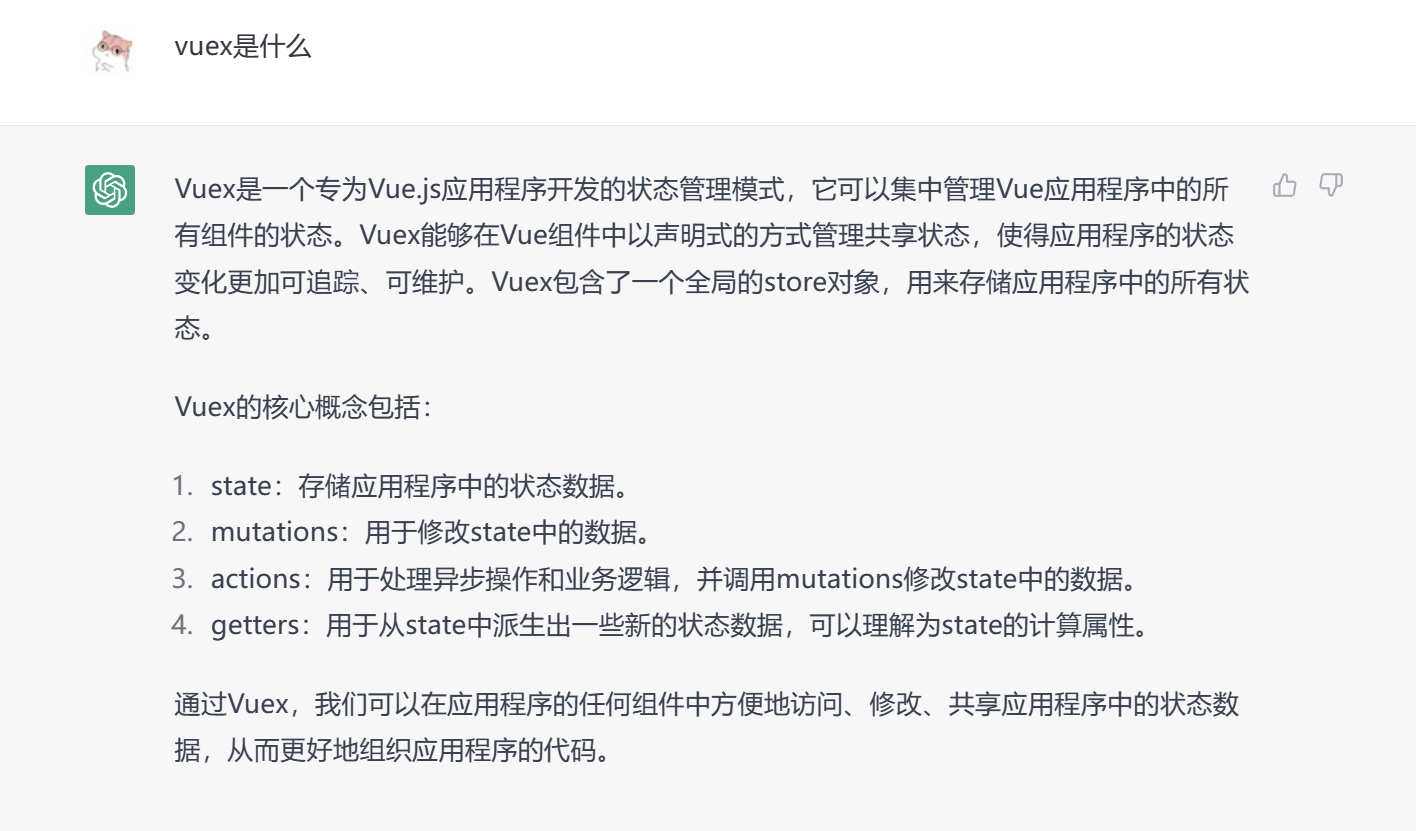
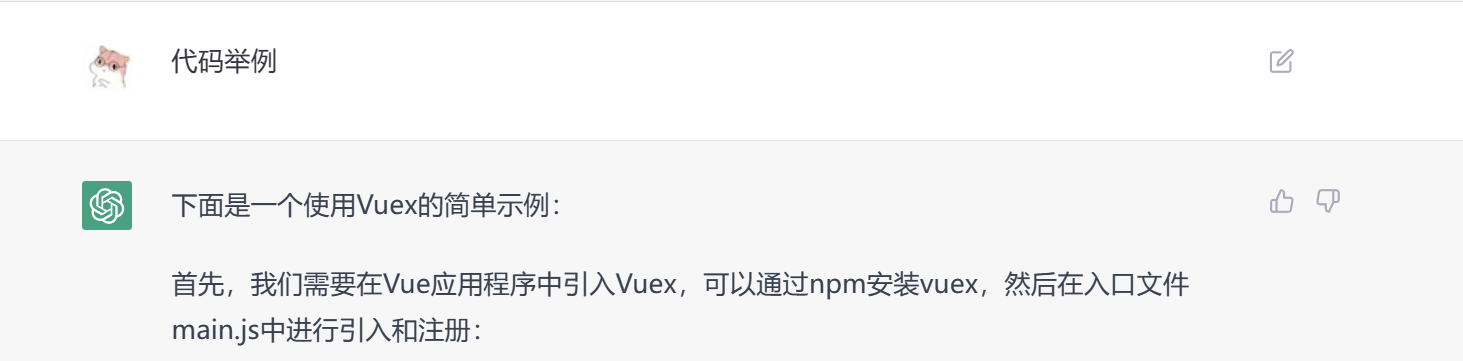
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
const store = new Vuex.Store({
state: {
count: 0
},
mutations: {
increment(state) {
state.count++
}
},
actions: {
incrementAsync(context) {
setTimeout(() => {
context.commit('increment')
}, 1000)
}
},
getters: {
doubleCount(state) {
return state.count * 2
}
}
})
new Vue({
el: '#app',
store,
template: `
<div>
<p>Count: {{ $store.state.count }}</p>
<p>Double Count: {{ $store.getters.doubleCount }}</p>
<button @click="$store.commit('increment')">Increment</button>
<button @click="$store.dispatch('incrementAsync')">Increment Async</button>
</div>
`
})
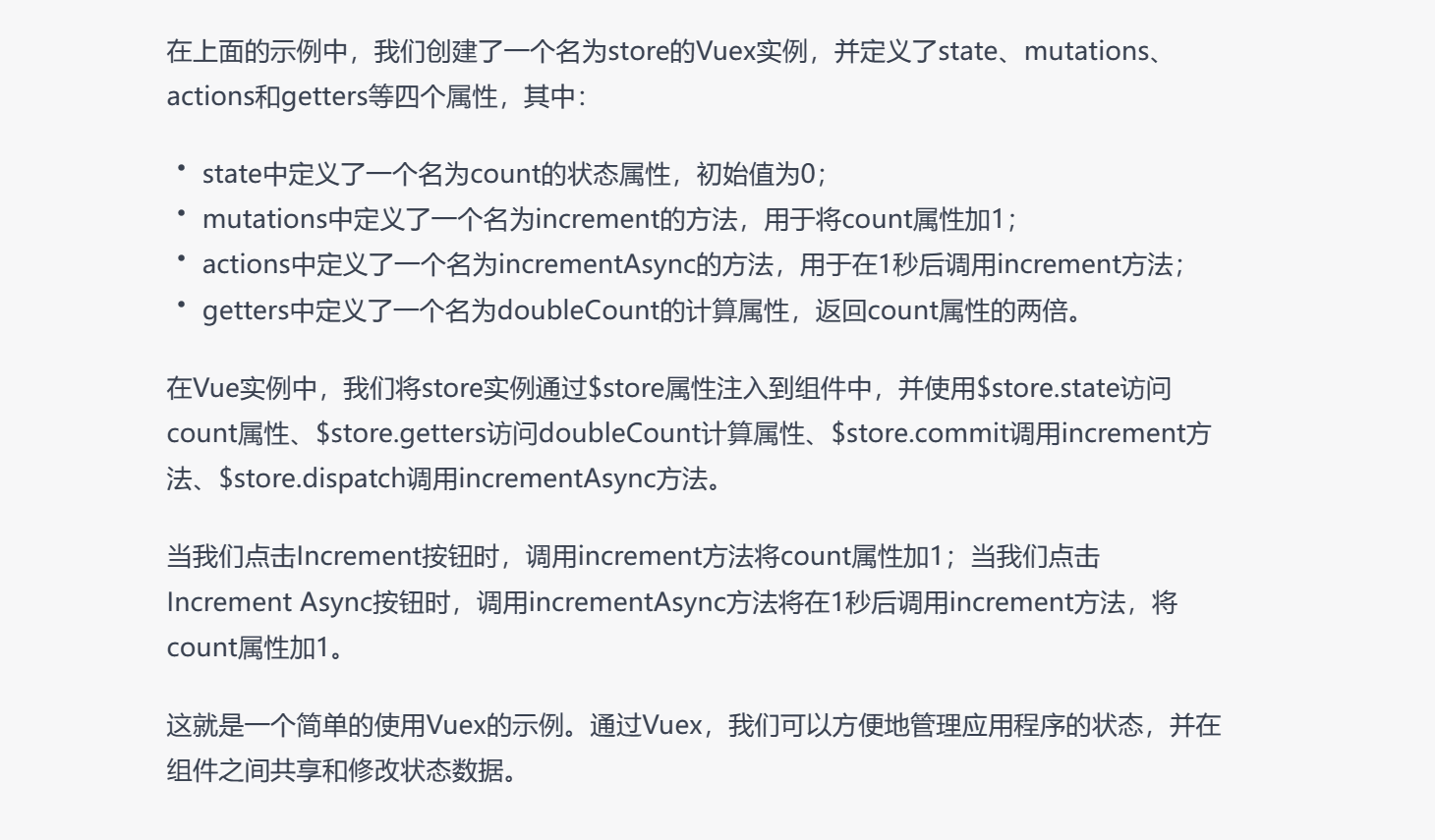
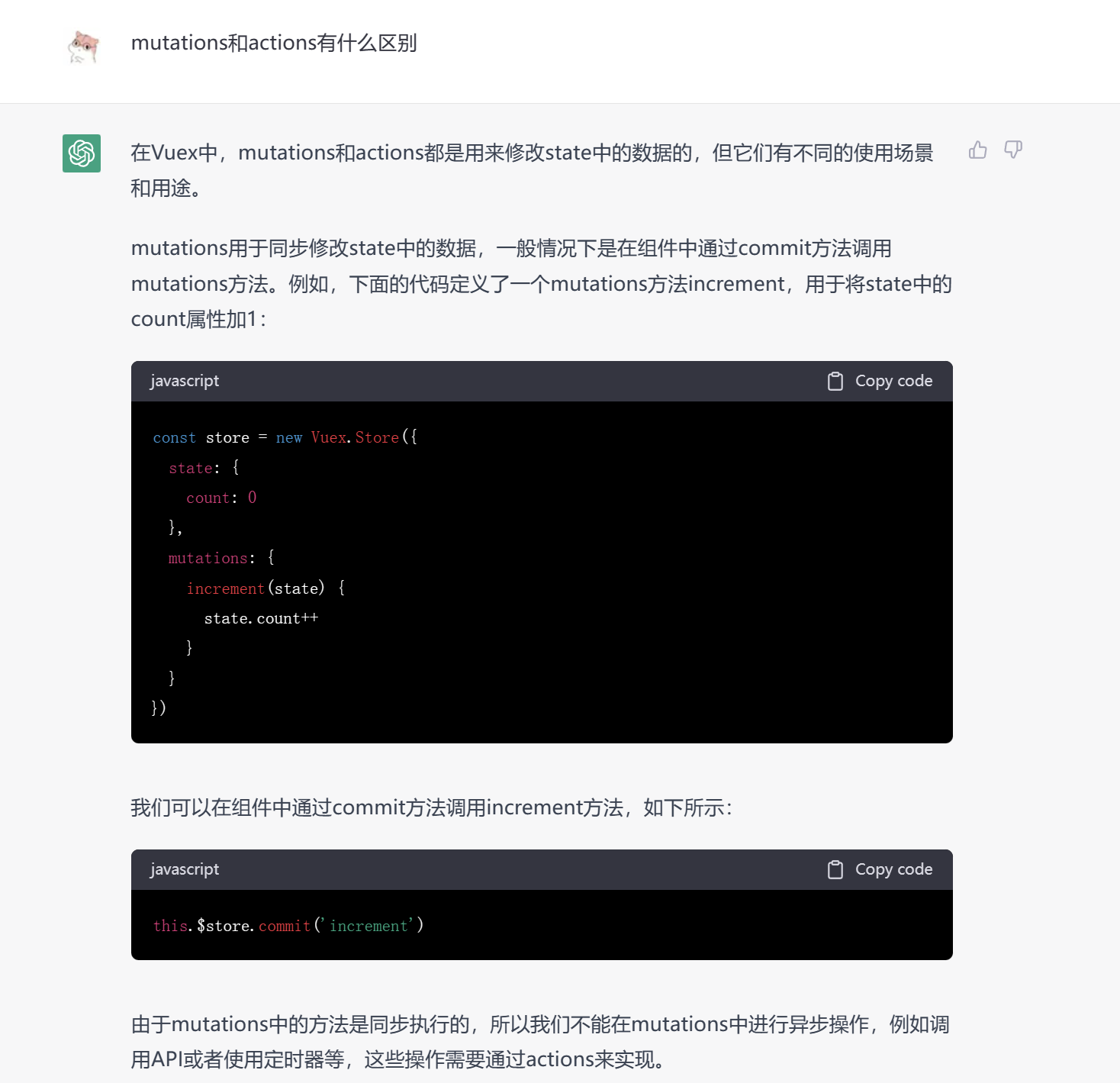
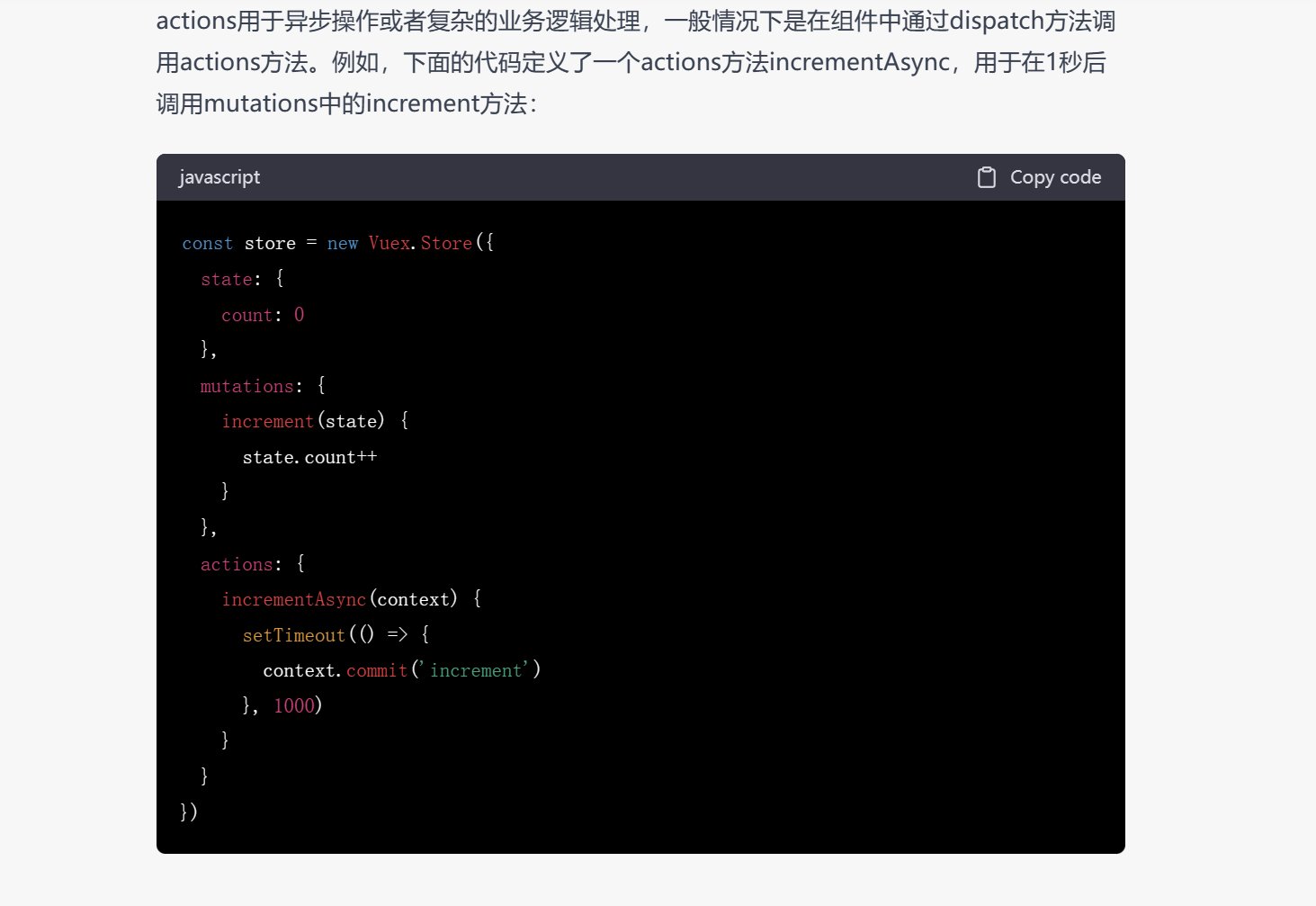
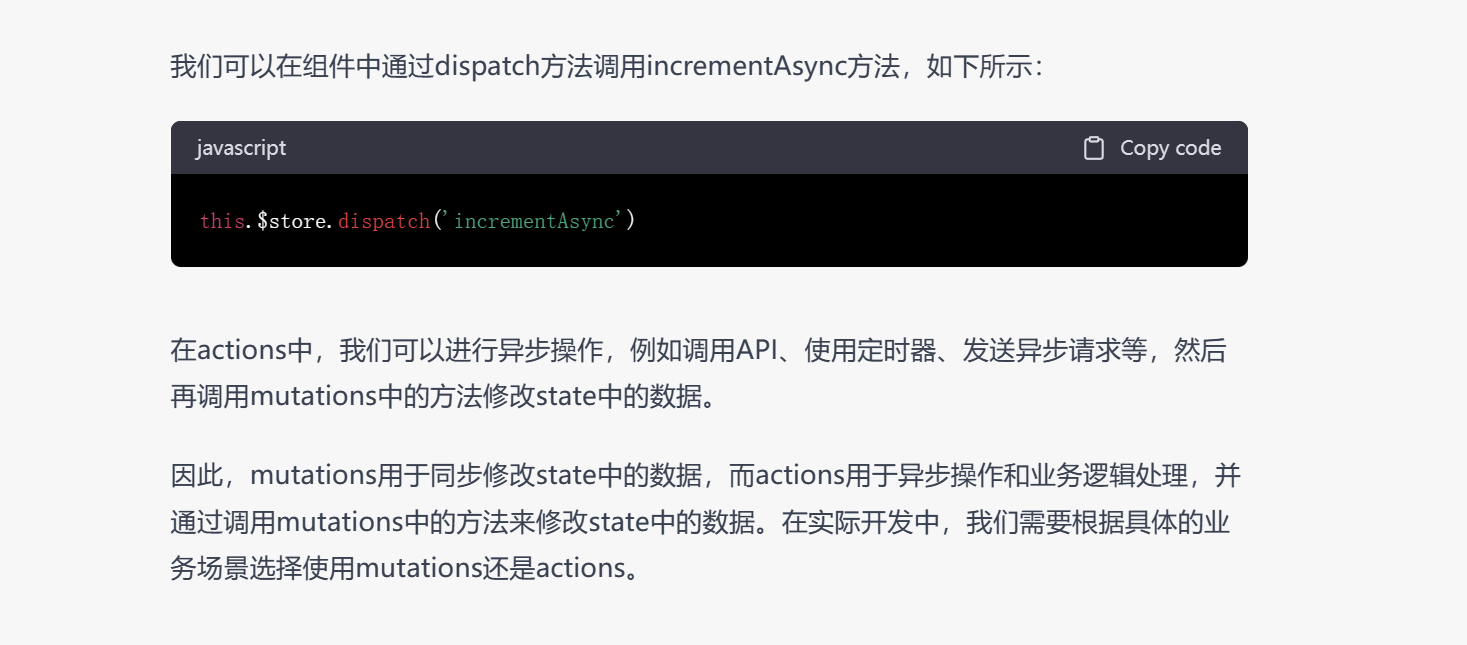

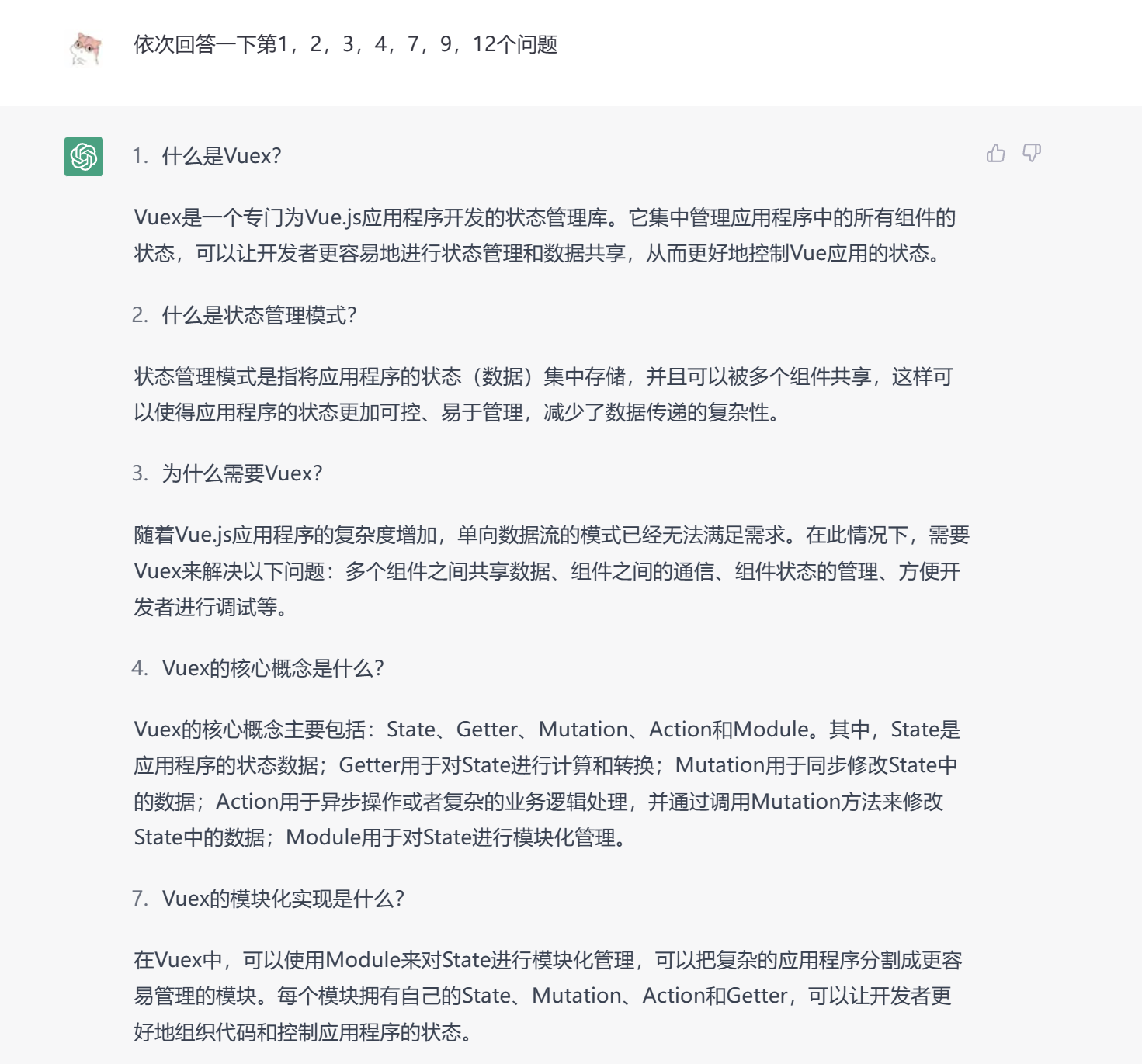
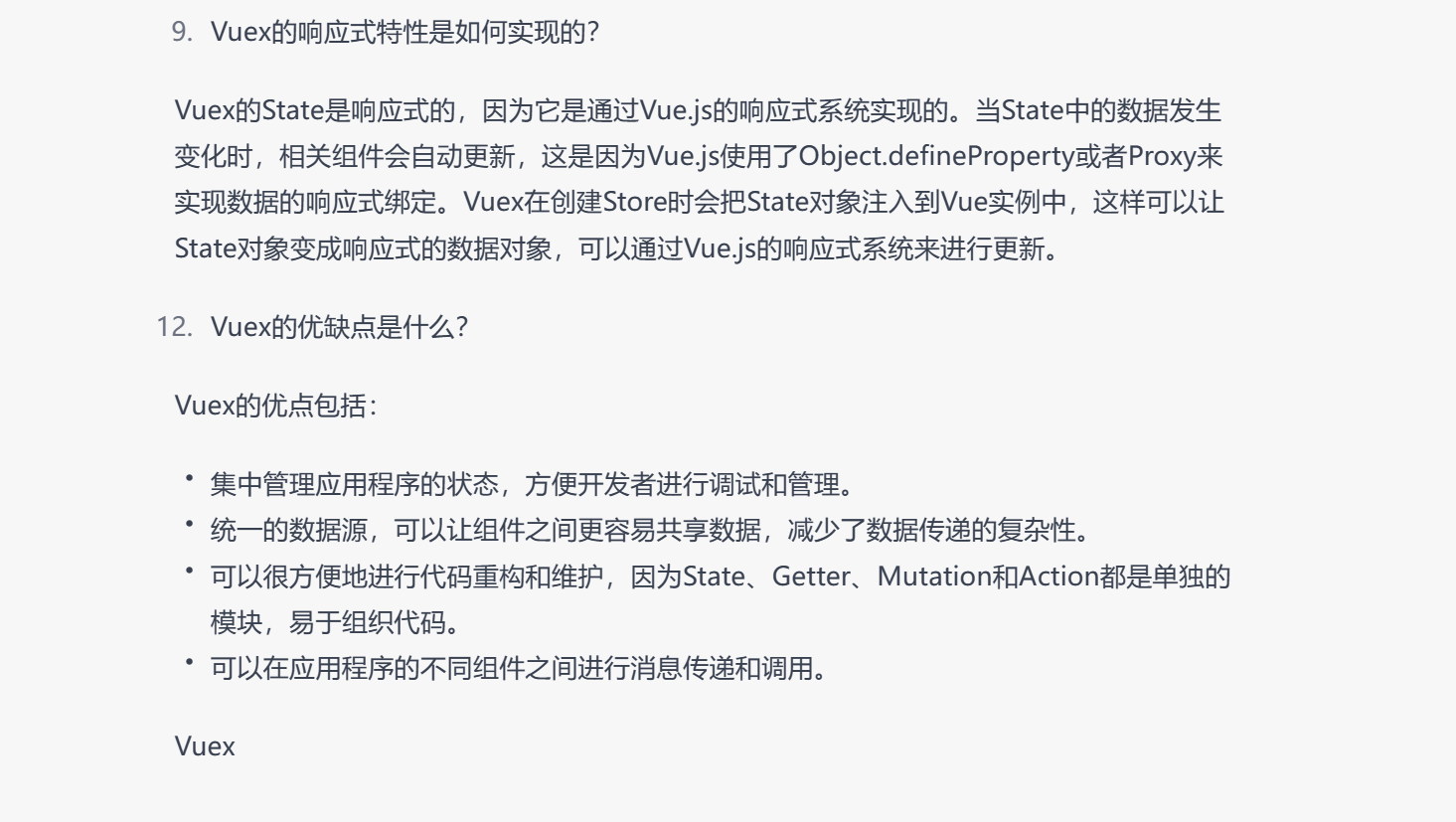

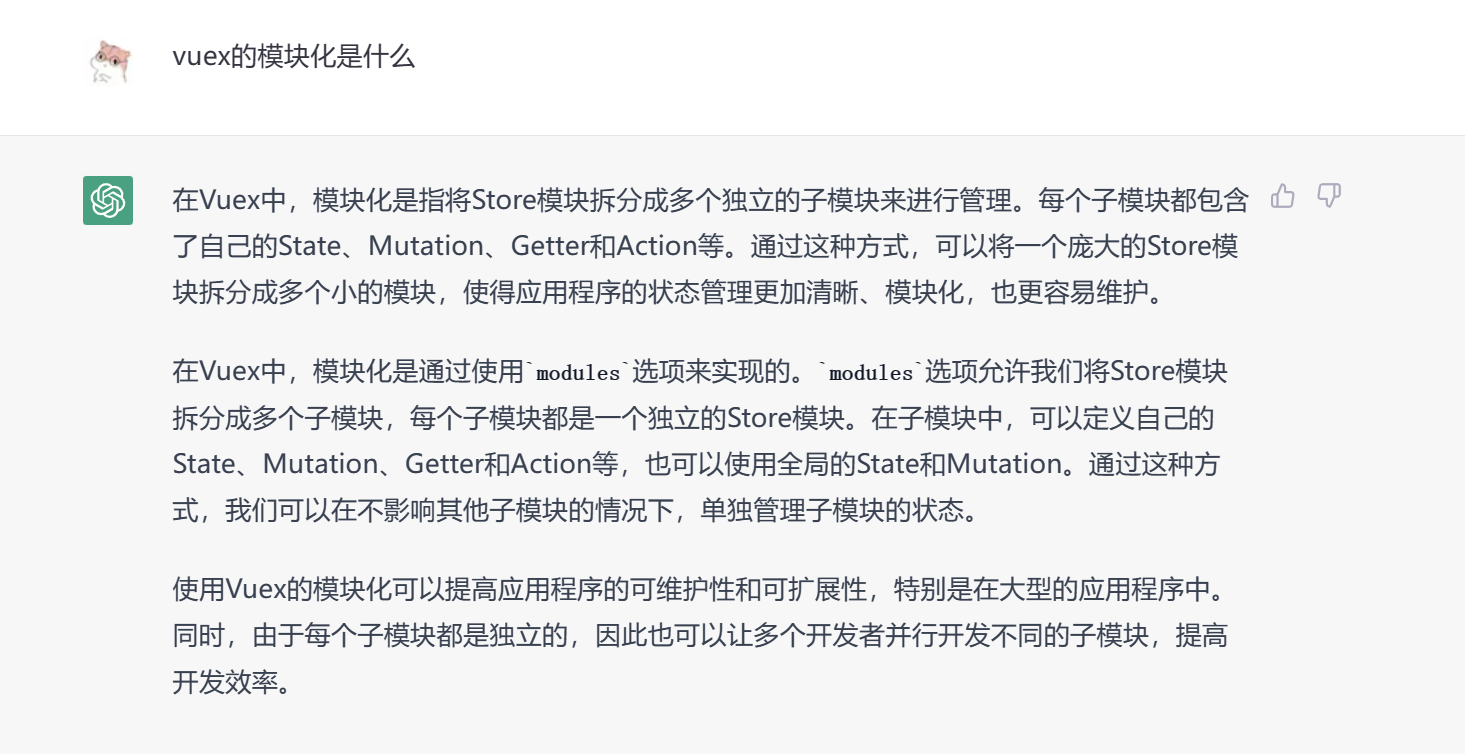

const products = {
state: {
productList: [],
loading: false,
error: null
},
mutations: {
setProductList(state, products) {
state.productList = products
},
setLoading(state, loading) {
state.loading = loading
},
setError(state, error) {
state.error = error
}
},
actions: {
fetchProducts({ commit }) {
commit('setLoading', true)
fetch('/api/products')
.then(response => response.json())
.then(products => {
commit('setProductList', products)
commit('setLoading', false)
})
.catch(error => {
commit('setError', error)
commit('setLoading', false)
})
}
},
getters: {
getProductById: (state) => (id) => {
return state.productList.find(product => product.id === id)
}
}
}

const cart = {
state: {
cartList: [],
total: 0
},
mutations: {
setCartList(state, cartList) {
state.cartList = cartList
},
setTotal(state, total) {
state.total = total
}
},
actions: {
fetchCart({ commit }) {
fetch('/api/cart')
.then(response => response.json())
.then(cart => {
commit('setCartList', cart.cartList)
commit('setTotal', cart.total)
})
}
},
getters: {
getCartById: (state) => (id) => {
return state.cartList.find(item => item.id === id)
}
}
}

const orders = {
state: {
orderList: [],
loading: false,
error: null
},
mutations: {
setOrderList(state, orders) {
state.orderList = orders
},
setLoading(state, loading) {
state.loading = loading
},
setError(state, error) {
state.error = error
}
},
actions: {
fetchOrders({ commit }) {
commit('setLoading', true)
fetch('/api/orders')
.then(response => response.json())
.then(orders => {
commit('setOrderList', orders)
commit('setLoading', false)
})
.catch(error => {
commit('setError', error)
commit('setLoading', false)
})
}
},
getters: {
getOrderById: (state) => (id) => {
return state.orderList.find(order => order.id === id)
}
}
}

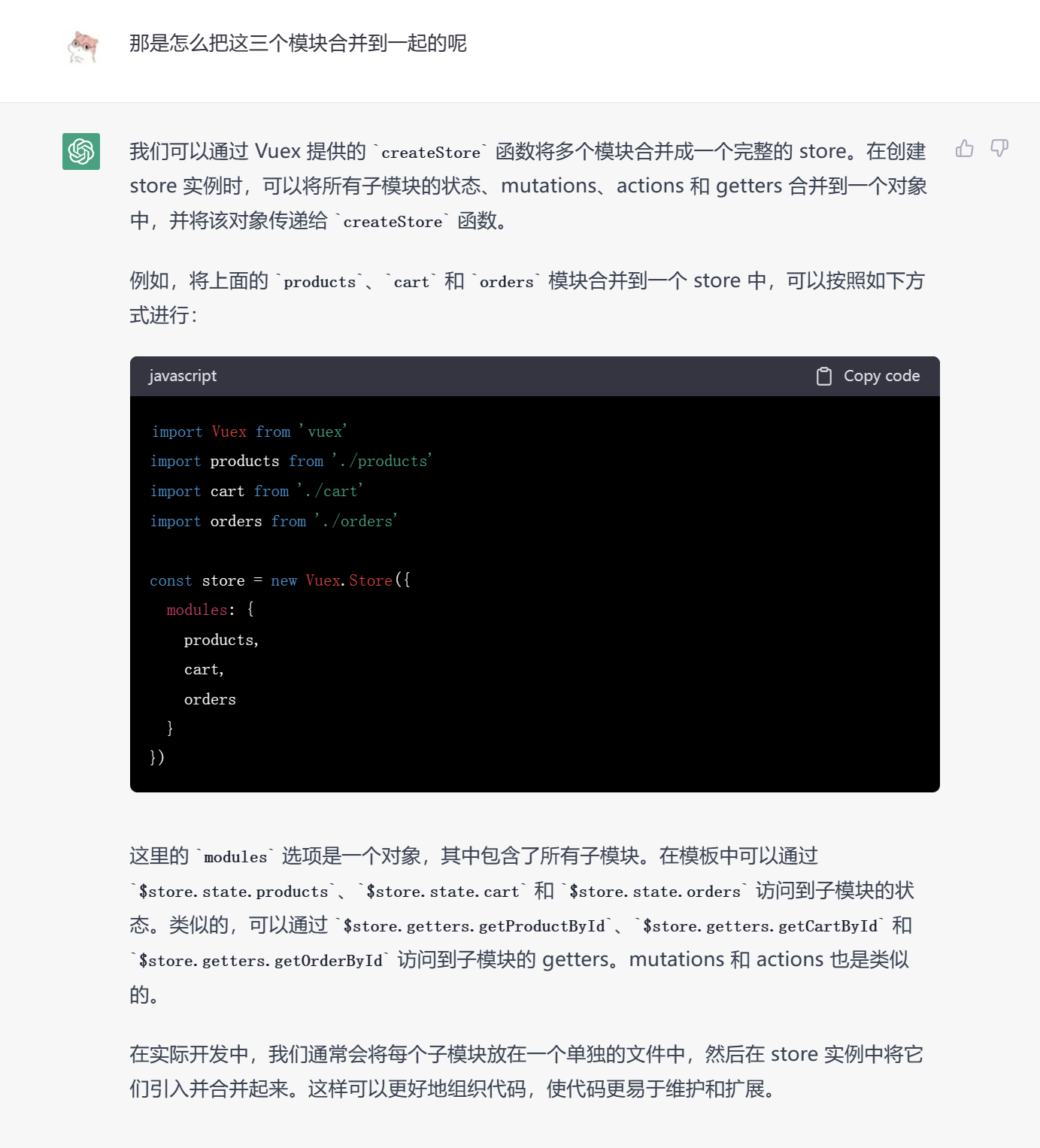